dancing flower
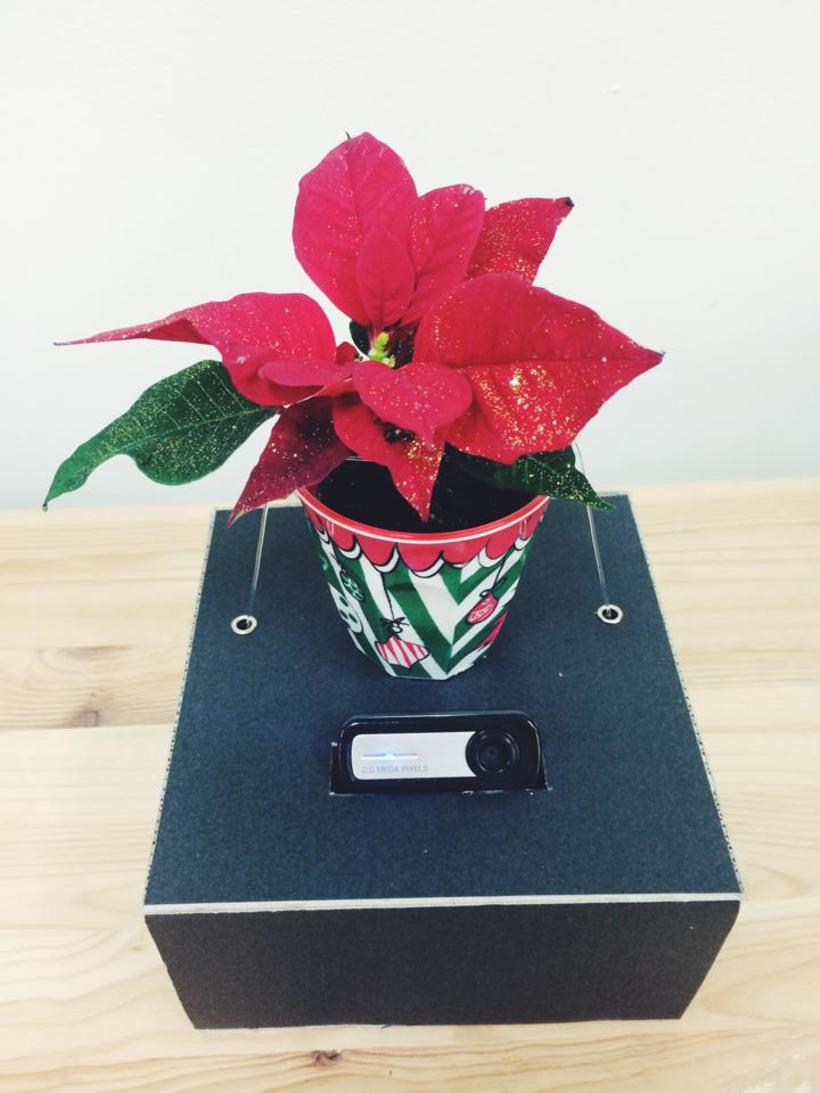
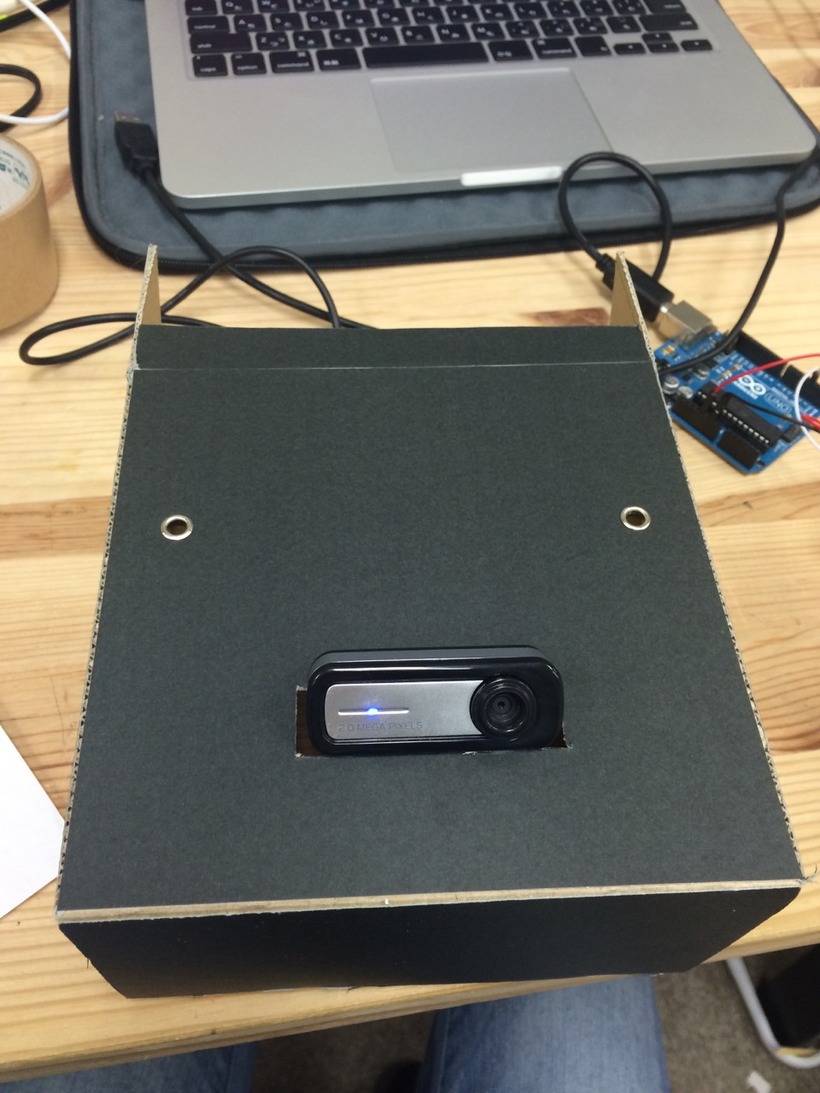
//Risa Hiyama
// a template for receiving face tracking osc messages from
// Kyle McDonald's FaceOSC https://github.com/kylemcdonald/ofxFaceTracker
//
//
// adapted from from Greg Borenstein's 2011 example
// http://www.gregborenstein.com/
// https://gist.github.com/1603230
import oscP5.*;
OscP5 oscP5;
import processing.serial.*;
import cc.arduino.*;
Arduino arduino;
//int ledPinRed = 13;
//int ledPinGreen = 12;
int servoPin0 = 3; // Control pin for servo motor
// num faces found
int found;
// pose
float poseScale;
PVector posePosition = new PVector();
PVector poseOrientation = new PVector();
// gesture
float mouthHeight;
float mouthWidth;
float eyeLeft;
float eyeRight;
float eyebrowLeft;
float eyebrowRight;
float jaw;
float nostrils;
void setup() {
minim = new Minim(this);
player = minim.loadFile("Giggles.mp3");
//player = minim.loadFile("Bells.mp3");
arduino = new Arduino(this, "/dev/tty.usbmodem1411");
arduino.pinMode(servoPin0, Arduino.OUTPUT);
arduino.analogWrite(servoPin0, 0);
delay(600);
//setting up general rule
size(640, 480);
noCursor();
frameRate(30);
textSize( 24 );
//faceOSC function
oscP5 = new OscP5(this, 8338);
oscP5.plug(this, "found", "/found");
oscP5.plug(this, "poseScale", "/pose/scale");
oscP5.plug(this, "posePosition", "/pose/position");
oscP5.plug(this, "poseOrientation", "/pose/orientation");
oscP5.plug(this, "mouthWidthReceived", "/gesture/mouth/width");
oscP5.plug(this, "mouthHeightReceived", "/gesture/mouth/height");
oscP5.plug(this, "eyeLeftReceived", "/gesture/eye/left");
oscP5.plug(this, "eyeRightReceived", "/gesture/eye/right");
oscP5.plug(this, "eyebrowLeftReceived", "/gesture/eyebrow/left");
oscP5.plug(this, "eyebrowRightReceived", "/gesture/eyebrow/right");
oscP5.plug(this, "jawReceived", "/gesture/jaw");
oscP5.plug(this, "nostrilsReceived", "/gesture/nostrils");
}
void draw() {
stroke(0);
background(255);
drawFace();
if ( mouthWidth >14 ) {//smile
//player.play(); //再生
player.rewind(); //再生が終わったら巻き戻しておく
arduino.analogWrite(servoPin0, 200);
delay(1000);
arduino.analogWrite(servoPin0, 270);
delay(1000);
fill(255, 192-mouthWidth, 203-mouthWidth);
ellipse(posePosition.x-80, posePosition.y+eyeLeft * -13, 50, 40);
ellipse(posePosition.x+80, posePosition.y+eyeRight * -13, 50, 40);
} else {
}
}
void drawFace() {
if (found > 0) {
noFill();
ellipse(posePosition.x, posePosition.y - 80, 290, 290);
ellipse(posePosition.x-60, posePosition.y+eyeLeft * -27, 40, 21);
ellipse(posePosition.x+60, posePosition.y+eyeRight * -27, 40, 21);
noFill();
ellipse(posePosition.x, posePosition.y+10, mouthWidth* 9, mouthHeight * 9);
ellipse(posePosition.x-15, posePosition.y+nostrils * - 6, 15, 9);
ellipse(posePosition.x+15, posePosition.y+nostrils * - 6, 15, 9);
rectMode(CENTER);
fill(0);
rect(posePosition.x-60, posePosition.y+eyebrowLeft * -15, 75, 15);
rect(posePosition.x+60, posePosition.y+eyebrowRight * -15, 75, 15);
}
}
public void found(int i) {
println("found: " + i);
found = i;
}
public void poseScale(float s) {
println("scale: " + s);
poseScale = s;
}
public void posePosition(float x, float y) {
println("pose position\tX: " + x + " Y: " + y );
posePosition.set(x, y, 0);
}
public void poseOrientation(float x, float y, float z) {
println("pose orientation\tX: " + x + " Y: " + y + " Z: " + z);
poseOrientation.set(x, y, z);
}
public void mouthWidthReceived(float w) {
println("mouth Width: " + w);
mouthWidth = w;
}
public void mouthHeightReceived(float h) {
println("mouth height: " + h);
mouthHeight = h;
}
public void eyeLeftReceived(float f) {
println("eye left: " + f);
eyeLeft = f;
}
public void eyeRightReceived(float f) {
println("eye right: " + f);
eyeRight = f;
}
public void eyebrowLeftReceived(float f) {
println("eyebrow left: " + f);
eyebrowLeft = f;
}
public void eyebrowRightReceived(float f) {
println("eyebrow right: " + f);
eyebrowRight = f;
}
public void jawReceived(float f) {
println("jaw: " + f);
jaw = f;
}
public void nostrilsReceived(float f) {
println("nostrils: " + f);
nostrils = f;
}
// all other OSC messages end up here
void oscEvent(OscMessage m) {
if (m.isPlugged() == false) {
println("UNPLUGGED: " + m);
}
}